// // SPTakeLocationListCell.m // SpecialtyProject // // Created by xxxx on 20xx/x/x. // Copyright © 20xx年 xxxx. All rights reserved. // #import "SPTakeLocationListCell.h" @implementation SPTakeLocationListCell - (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier { self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]; if(self){ _userNameAndPhoneLabel = [UILabel new]; [_userNameAndPhoneLabel setTranslatesAutoresizingMaskIntoConstraints:NO]; _userNameAndPhoneLabel.backgroundColor = [UIColor clearColor]; [_userNameAndPhoneLabel setTextColor:[UIColor blackColor]]; [_userNameAndPhoneLabel setFont:[CWFont setSysFontSize:ScaleForLengthWith2(28.0)]]; [self.contentView addSubview:_userNameAndPhoneLabel]; // _editOperateButton = [UILabel new]; _editOperateButton.userInteractionEnabled = YES; [_editOperateButton setTranslatesAutoresizingMaskIntoConstraints:NO]; _editOperateButton.backgroundColor = [UIColor clearColor]; [_editOperateButton setTextColor:[UIColor grayColor]]; [_editOperateButton setTextAlignment:(NSTextAlignmentCenter)]; _editOperateButton.text = @"\U0000e61c"; [_editOperateButton setFont:[CWFont setCWFontSize:ScaleForLengthWith2(46.0)]]; [self.contentView addSubview:_editOperateButton]; UITapGestureRecognizer *singTap = [[UITapGestureRecognizer alloc]initWithTarget:self action:@selector(editAction:)]; singTap.numberOfTapsRequired = 1; [_editOperateButton addGestureRecognizer:singTap]; // _userLocationlabel = [UILabel new]; [_userLocationlabel setTranslatesAutoresizingMaskIntoConstraints:NO]; _userLocationlabel.backgroundColor = [UIColor clearColor]; [_userLocationlabel setTextColor:[UIColor darkGrayColor]]; [_userLocationlabel setFont:[CWFont setSysFontSize:ScaleForLengthWith2(24.0)]]; _userLocationlabel.numberOfLines = 0; _userLocationlabel.opaque = NO; _userLocationlabel.lineBreakMode = 0; [self.contentView addSubview:_userLocationlabel]; // _userDefaultIconLabel = [UILabel new]; [_userDefaultIconLabel setTranslatesAutoresizingMaskIntoConstraints:NO]; _userDefaultIconLabel.backgroundColor = [UIColor orangeColor]; _userDefaultIconLabel.clipsToBounds = YES; [_userDefaultIconLabel setTextAlignment:(NSTextAlignmentCenter)]; _userDefaultIconLabel.text = @"默认"; _userDefaultIconLabel.layer.cornerRadius = 2.0; [_userDefaultIconLabel setTextColor:[UIColor blackColor]]; [_userDefaultIconLabel setFont:[CWFont setSysFontSize:ScaleForLengthWith2(24.0)]]; [self.contentView addSubview:_userDefaultIconLabel]; // _horLineView = [UIView new]; [_horLineView setTranslatesAutoresizingMaskIntoConstraints:NO]; _horLineView.backgroundColor = [UIColor groupTableViewBackgroundColor]; [self.contentView addSubview:_horLineView]; // _verLineView = [UIView new]; [_verLineView setTranslatesAutoresizingMaskIntoConstraints:NO]; _verLineView.backgroundColor = [UIColor groupTableViewBackgroundColor]; [self.contentView addSubview:_verLineView]; // NSArray *lineHotConstraint = [NSLayoutConstraint constraintsWithVisualFormat:@"H:|-(verSpace)-[_horLineView]-(verSpace)-|" options:0 metrics:@{@"verSpace":@(ScaleForLengthWith2(20.0))} views:NSDictionaryOfVariableBindings(_horLineView)]; [self.contentView addConstraints:lineHotConstraint]; NSArray *nameVerConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"V:|-(==nameAndPhoneTopSpace)-[_userNameAndPhoneLabel(==nameAndPhoneHeight)]-(locationAndNameSpace)-[_userLocationlabel]-(locationToHerLineSpace)-[_horLineView(==horLineHeight)]|" options:0 metrics:@{@"nameAndPhoneTopSpace":@(ScaleForLengthWith2(40.0)),@"nameAndPhoneHeight":@(ScaleForLengthWith2(38.0)),@"locationAndNameSpace":@(ScaleForLengthWith2(10.0)),@"locationToHerLineSpace":@(ScaleForLengthWith2(35.0)),@"horLineHeight":@(ScaleForLengthWith2(ProjectDefaultLineHeight))} views:NSDictionaryOfVariableBindings(_userNameAndPhoneLabel,_userLocationlabel,_horLineView)]; [self.contentView addConstraints:nameVerConstraints]; // NSArray *herConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"H:|-(nameLeftSpace)-[_userNameAndPhoneLabel]-(nameToDefaultSpace)-[_userDefaultIconLabel(==defaultIconLabelWidth)]-(defaultIconLabelToVerLineSpace)-[_verLineView(==verLineWidth)]-[_editOperateButton(==editButtonWidth)]-(editButtonRightSpace)-|" options:0 metrics:@{@"nameLeftSpace":@(ScaleForLengthWith2(30.0)),@"nameToDefaultSpace":@(ScaleForLengthWith2(20.0)),@"defaultIconLabelWidth":@(ScaleForLengthWith2(72.0)),@"defaultIconLabelToVerLineSpace":@(ScaleForLengthWith2(14.0)),@"verLineWidth":@(ScaleForLengthWith2(ProjectDefaultLineHeight)),@"editButtonWidth":@(ScaleForLengthWith2(88.0)),@"editButtonRightSpace":@(ScaleForLengthWith2(20.0))} views:NSDictionaryOfVariableBindings(_userNameAndPhoneLabel,_userDefaultIconLabel,_userLocationlabel,_horLineView,_verLineView,_editOperateButton)]; [self.contentView addConstraints:herConstraints]; //default icon top and size NSLayoutConstraint *defaultIconTopConstraint = [NSLayoutConstraint constraintWithItem:_userDefaultIconLabel attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:self.contentView attribute:NSLayoutAttributeTop multiplier:1.0 constant:ScaleForLengthWith2(66.0)]; [self.contentView addConstraint:defaultIconTopConstraint]; NSLayoutConstraint *defaultIconSizeConstraint = [NSLayoutConstraint constraintWithItem:_userDefaultIconLabel attribute:NSLayoutAttributeHeight relatedBy:NSLayoutRelationEqual toItem:nil attribute:NSLayoutAttributeNotAnAttribute multiplier:1.0 constant:ScaleForLengthWith2(38.0)]; [self.contentView addConstraint:defaultIconSizeConstraint]; // NSArray *verLineTopConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"V:|-(verLineTopAndBottomSpace)-[_verLineView]-(verLineTopAndBottomSpace)-|" options:0 metrics:@{@"verLineTopAndBottomSpace":@(ScaleForLengthWith2(26.0))} views:NSDictionaryOfVariableBindings(_verLineView)]; [self.contentView addConstraints:verLineTopConstraints]; // NSArray *editButtonTopConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"V:|[_editOperateButton]-(editButtonBottomSpace)-|" options:0 metrics:@{@"editButtonBottomSpace":@(ProjectDefaultLineHeight)} views:NSDictionaryOfVariableBindings(_editOperateButton)]; [self.contentView addConstraints:editButtonTopConstraints]; //name and phone left and right NSLayoutConstraint *nameLeftConstraint = [NSLayoutConstraint constraintWithItem:_userNameAndPhoneLabel attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.contentView attribute:NSLayoutAttributeLeft multiplier:1.0 constant:ScaleForLengthWith2(30.0)]; [self.contentView addConstraint:nameLeftConstraint]; NSLayoutConstraint *nameRightConstraint = [NSLayoutConstraint constraintWithItem:_verLineView attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:_userNameAndPhoneLabel attribute:NSLayoutAttributeRight multiplier:1.0 constant:ScaleForLengthWith2(20.0)]; [self.contentView addConstraint:nameRightConstraint]; //name and phone left and right NSLayoutConstraint *locationLeftConstraint = [NSLayoutConstraint constraintWithItem:_userLocationlabel attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.contentView attribute:NSLayoutAttributeLeft multiplier:1.0 constant:ScaleForLengthWith2(30.0)]; [self.contentView addConstraint:locationLeftConstraint]; NSLayoutConstraint *locationRightConstraint = [NSLayoutConstraint constraintWithItem:_userDefaultIconLabel attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:_userLocationlabel attribute:NSLayoutAttributeRight multiplier:1.0 constant:ScaleForLengthWith2(20.0)]; [self.contentView addConstraint:locationRightConstraint]; // } return self; } - (void)configLocationDataWithModel:(id)model { _userNameAndPhoneLabel.text = @"王小二 133542222222"; //_userLocationlabel.text = @"北京市昌平区天通苑老一区1单元102"; } - (void)editAction:(UITapGestureRecognizer *)tap { tap.view.alpha = 0.5; [UIView animateWithDuration:0.2 animations:^{ tap.view.alpha = 1.0; } completion:^(BOOL finished) { if(_delegate && [_delegate respondsToSelector:@selector(takeLocationListCellClickEditWithCell:)]){ [_delegate takeLocationListCellClickEditWithCell:self]; } }]; } @end
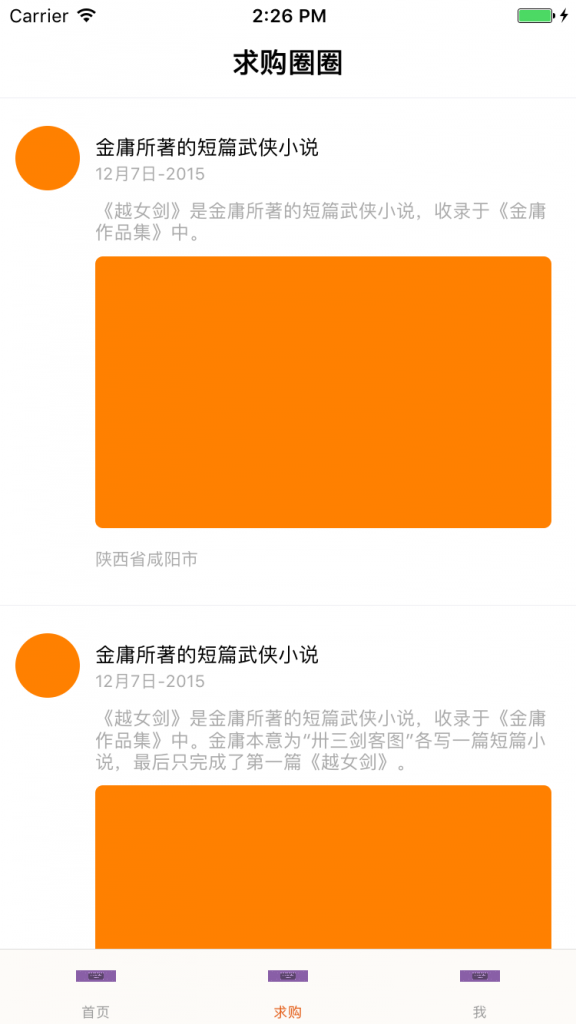
// // SPTakeLocationListCell.m // SpecialtyProject // // Created by xxxx on 20xx/x/x. // Copyright © 20xx年 xxxx. All rights reserved. // #import "SPTakeLocationListCell.h" @implementation SPTakeLocationListCell - (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier { self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]; if(self){ _userNameAndPhoneLabel = [UILabel new]; [_userNameAndPhoneLabel setTranslatesAutoresizingMaskIntoConstraints:NO]; _userNameAndPhoneLabel.backgroundColor = [UIColor clearColor]; [_userNameAndPhoneLabel setTextColor:[UIColor blackColor]]; [_userNameAndPhoneLabel setFont:[CWFont setSysFontSize:ScaleForLengthWith2(28.0)]]; [self.contentView addSubview:_userNameAndPhoneLabel]; // _editOperateButton = [UILabel new]; _editOperateButton.userInteractionEnabled = YES; [_editOperateButton setTranslatesAutoresizingMaskIntoConstraints:NO]; _editOperateButton.backgroundColor = [UIColor clearColor]; [_editOperateButton setTextColor:[UIColor grayColor]]; [_editOperateButton setTextAlignment:(NSTextAlignmentCenter)]; _editOperateButton.text = @"\U0000e61c"; [_editOperateButton setFont:[CWFont setCWFontSize:ScaleForLengthWith2(46.0)]]; [self.contentView addSubview:_editOperateButton]; UITapGestureRecognizer *singTap = [[UITapGestureRecognizer alloc]initWithTarget:self action:@selector(editAction:)]; singTap.numberOfTapsRequired = 1; [_editOperateButton addGestureRecognizer:singTap]; // _userLocationlabel = [UILabel new]; [_userLocationlabel setTranslatesAutoresizingMaskIntoConstraints:NO]; _userLocationlabel.backgroundColor = [UIColor clearColor]; [_userLocationlabel setTextColor:[UIColor darkGrayColor]]; [_userLocationlabel setFont:[CWFont setSysFontSize:ScaleForLengthWith2(24.0)]]; _userLocationlabel.numberOfLines = 0; _userLocationlabel.opaque = NO; _userLocationlabel.lineBreakMode = 0; [self.contentView addSubview:_userLocationlabel]; // _userDefaultIconLabel = [UILabel new]; [_userDefaultIconLabel setTranslatesAutoresizingMaskIntoConstraints:NO]; _userDefaultIconLabel.backgroundColor = [UIColor orangeColor]; _userDefaultIconLabel.clipsToBounds = YES; [_userDefaultIconLabel setTextAlignment:(NSTextAlignmentCenter)]; _userDefaultIconLabel.text = @"默认"; _userDefaultIconLabel.layer.cornerRadius = 2.0; [_userDefaultIconLabel setTextColor:[UIColor blackColor]]; [_userDefaultIconLabel setFont:[CWFont setSysFontSize:ScaleForLengthWith2(24.0)]]; [self.contentView addSubview:_userDefaultIconLabel]; // _horLineView = [UIView new]; [_horLineView setTranslatesAutoresizingMaskIntoConstraints:NO]; _horLineView.backgroundColor = [UIColor groupTableViewBackgroundColor]; [self.contentView addSubview:_horLineView]; // _verLineView = [UIView new]; [_verLineView setTranslatesAutoresizingMaskIntoConstraints:NO]; _verLineView.backgroundColor = [UIColor groupTableViewBackgroundColor]; [self.contentView addSubview:_verLineView]; // NSArray *lineHotConstraint = [NSLayoutConstraint constraintsWithVisualFormat:@"H:|-(verSpace)-[_horLineView]-(verSpace)-|" options:0 metrics:@{@"verSpace":@(ScaleForLengthWith2(20.0))} views:NSDictionaryOfVariableBindings(_horLineView)]; [self.contentView addConstraints:lineHotConstraint]; NSArray *nameVerConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"V:|-(==nameAndPhoneTopSpace)-[_userNameAndPhoneLabel(==nameAndPhoneHeight)]-(locationAndNameSpace)-[_userLocationlabel]-(locationToHerLineSpace)-[_horLineView(==horLineHeight)]|" options:0 metrics:@{@"nameAndPhoneTopSpace":@(ScaleForLengthWith2(40.0)),@"nameAndPhoneHeight":@(ScaleForLengthWith2(38.0)),@"locationAndNameSpace":@(ScaleForLengthWith2(10.0)),@"locationToHerLineSpace":@(ScaleForLengthWith2(35.0)),@"horLineHeight":@(ScaleForLengthWith2(ProjectDefaultLineHeight))} views:NSDictionaryOfVariableBindings(_userNameAndPhoneLabel,_userLocationlabel,_horLineView)]; [self.contentView addConstraints:nameVerConstraints]; // NSArray *herConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"H:|-(nameLeftSpace)-[_userNameAndPhoneLabel]-(nameToDefaultSpace)-[_userDefaultIconLabel(==defaultIconLabelWidth)]-(defaultIconLabelToVerLineSpace)-[_verLineView(==verLineWidth)]-[_editOperateButton(==editButtonWidth)]-(editButtonRightSpace)-|" options:0 metrics:@{@"nameLeftSpace":@(ScaleForLengthWith2(30.0)),@"nameToDefaultSpace":@(ScaleForLengthWith2(20.0)),@"defaultIconLabelWidth":@(ScaleForLengthWith2(72.0)),@"defaultIconLabelToVerLineSpace":@(ScaleForLengthWith2(14.0)),@"verLineWidth":@(ScaleForLengthWith2(ProjectDefaultLineHeight)),@"editButtonWidth":@(ScaleForLengthWith2(88.0)),@"editButtonRightSpace":@(ScaleForLengthWith2(20.0))} views:NSDictionaryOfVariableBindings(_userNameAndPhoneLabel,_userDefaultIconLabel,_userLocationlabel,_horLineView,_verLineView,_editOperateButton)]; [self.contentView addConstraints:herConstraints]; //default icon top and size NSLayoutConstraint *defaultIconTopConstraint = [NSLayoutConstraint constraintWithItem:_userDefaultIconLabel attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:self.contentView attribute:NSLayoutAttributeTop multiplier:1.0 constant:ScaleForLengthWith2(66.0)]; [self.contentView addConstraint:defaultIconTopConstraint]; NSLayoutConstraint *defaultIconSizeConstraint = [NSLayoutConstraint constraintWithItem:_userDefaultIconLabel attribute:NSLayoutAttributeHeight relatedBy:NSLayoutRelationEqual toItem:nil attribute:NSLayoutAttributeNotAnAttribute multiplier:1.0 constant:ScaleForLengthWith2(38.0)]; [self.contentView addConstraint:defaultIconSizeConstraint]; // NSArray *verLineTopConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"V:|-(verLineTopAndBottomSpace)-[_verLineView]-(verLineTopAndBottomSpace)-|" options:0 metrics:@{@"verLineTopAndBottomSpace":@(ScaleForLengthWith2(26.0))} views:NSDictionaryOfVariableBindings(_verLineView)]; [self.contentView addConstraints:verLineTopConstraints]; // NSArray *editButtonTopConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"V:|[_editOperateButton]-(editButtonBottomSpace)-|" options:0 metrics:@{@"editButtonBottomSpace":@(ProjectDefaultLineHeight)} views:NSDictionaryOfVariableBindings(_editOperateButton)]; [self.contentView addConstraints:editButtonTopConstraints]; //name and phone left and right NSLayoutConstraint *nameLeftConstraint = [NSLayoutConstraint constraintWithItem:_userNameAndPhoneLabel attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.contentView attribute:NSLayoutAttributeLeft multiplier:1.0 constant:ScaleForLengthWith2(30.0)]; [self.contentView addConstraint:nameLeftConstraint]; NSLayoutConstraint *nameRightConstraint = [NSLayoutConstraint constraintWithItem:_verLineView attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:_userNameAndPhoneLabel attribute:NSLayoutAttributeRight multiplier:1.0 constant:ScaleForLengthWith2(20.0)]; [self.contentView addConstraint:nameRightConstraint]; //name and phone left and right NSLayoutConstraint *locationLeftConstraint = [NSLayoutConstraint constraintWithItem:_userLocationlabel attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.contentView attribute:NSLayoutAttributeLeft multiplier:1.0 constant:ScaleForLengthWith2(30.0)]; [self.contentView addConstraint:locationLeftConstraint]; NSLayoutConstraint *locationRightConstraint = [NSLayoutConstraint constraintWithItem:_userDefaultIconLabel attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:_userLocationlabel attribute:NSLayoutAttributeRight multiplier:1.0 constant:ScaleForLengthWith2(20.0)]; [self.contentView addConstraint:locationRightConstraint]; // } return self; } - (void)configLocationDataWithModel:(id)model { _userNameAndPhoneLabel.text = @"王小二 133542222222"; //_userLocationlabel.text = @"北京市昌平区天通苑老一区1单元102"; } - (void)editAction:(UITapGestureRecognizer *)tap { tap.view.alpha = 0.5; [UIView animateWithDuration:0.2 animations:^{ tap.view.alpha = 1.0; } completion:^(BOOL finished) { if(_delegate && [_delegate respondsToSelector:@selector(takeLocationListCellClickEditWithCell:)]){ [_delegate takeLocationListCellClickEditWithCell:self]; } }]; } @end
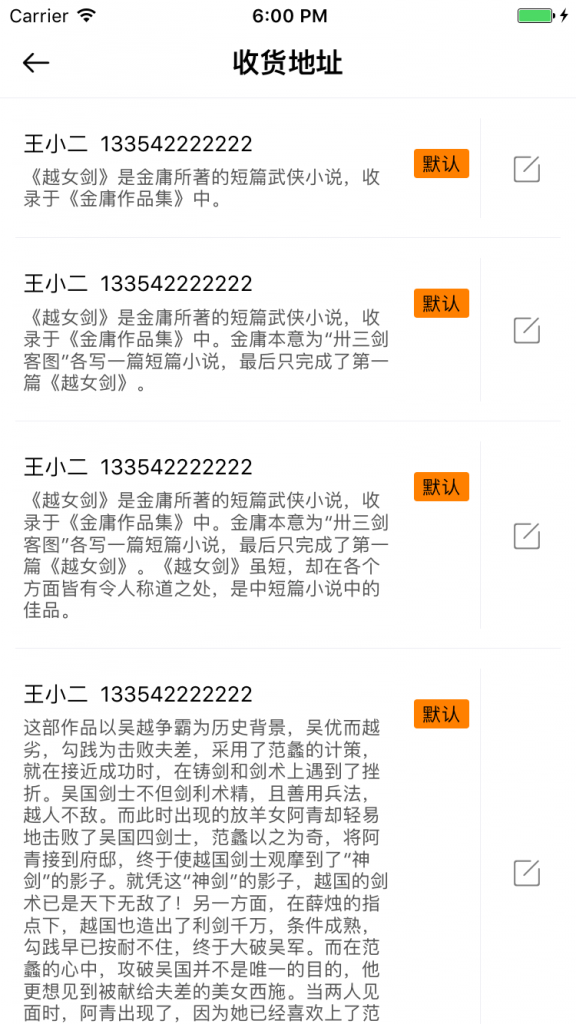